Exploring MIMIC-IV¶
Let’s explore the data in the MIMIC-IV Waveform Database.
Our objectives are to:
Become familiar with the file structure of the database.
Find out which signals are present in selected records and segments, and how long the signals last.
Search for records that contain signals of interest.
Load waveforms using the WFDB toolbox.
Plot one minute of signals from a segment of data
Look more closely at the shape of the PPG pulse waves
Resource: You can find out more about the MIMIC-IV Waveform Database here.
Setup¶
Specify the required Python packages¶
We’ll import the following:
sys: an essential python package
pathlib (well a particular function from pathlib, called Path)
import sys
from pathlib import Path
Specify a particular version of the WFDB Toolbox¶
wfdb: For this workshop we will be using version 4 of the WaveForm DataBase (WFDB) Toolbox package. The package contains tools for processing waveform data such as those found in MIMIC:
!pip install wfdb==4.1.0
import wfdb
Resource: You can find out more about the WFDB package here.
Now that we have imported these packages (i.e. toolboxes) we have a set of tools (functions) ready to use.
Specify the name of the MIMIC Waveform Database¶
Specify the name of the MIMIC IV Waveform Database on Physionet, which comes from the URL: https://physionet.org/content/mimic4wdb/0.1.0/
database_name = 'mimic4wdb/0.1.0'
Understanding the structure of the database¶
The waveform database is organized into “records”. Each record represents a single patient and roughly corresponds to a single ICU stay. Each record is stored in a separate subdirectory.
An example of the file structure is shown below. Here there are two patients (subject_id
10014354 and 10039708). There is one record (81739927) belonging to the first patient, and two records (83411188 and 85583557) belonging to the second. Each record is made of several segments (e.g. record 83411188 is composed of segments: 81739927_0000, 81739927_0001, etc).
waves
└── p100
├── p10014354
│ └── 81739927
│ ├── 81739927.dat
│ ├── 81739927_0000.hea
│ ├── 81739927_0001.hea
│ ├── 81739927_0001e.dat
│ ├── 81739927_0001r.dat
│ ├── 81739927_0002.hea
│ ├── 81739927_0002e.dat
│ ├── 81739927_0002p.dat
│ ├── 81739927_0002r.dat
│ ├── ...
│ └── 81739927n.csv.gz
├── ...
└── p10039708
├── 83411188
│ ├── 83411188.hea
│ ├── ...
│ └── 83411188n.csv.gz
└── 85583557
├── 85583557.hea
├── ...
└── 85583557n.csv.gz
Source: this content is adapted from the MIMIC WFDB Tutorials.
Identify the records in the database¶
Get a list of records¶
Use the
get_record_list
function from the WFDB toolbox to get a list of records in the database.
# each subject may be associated with multiple records
subjects = wfdb.get_record_list(database_name)
print(f"The '{database_name}' database contains data from {len(subjects)} subjects")
The 'mimic4wdb/0.1.0' database contains data from 198 subjects
# iterate the subjects to get a list of records
records = []
for subject in subjects:
studies = wfdb.get_record_list(f'{database_name}/{subject}')
for study in studies:
records.append(Path(f'{subject}{study}'))
print(f"Loaded {len(records)} records from the '{database_name}' database.")
Loaded 200 records from the 'mimic4wdb/0.1.0' database.
NB: There are 200 records for 198 subjects because two subjects had two records (we saw an example of this for subject 10039708 above).
Look at the records¶
Display the first few records
# format and print first five records
first_five_records = [str(x) for x in records[0:5]]
first_five_records = "\n - ".join(first_five_records)
print(f"First five records: \n - {first_five_records}")
print("""
Note the formatting of these records:
- intermediate directory ('p100' in this case)
- subject identifier (e.g. 'p10014354')
- record identifier (e.g. '81739927'
""")
First five records:
- waves/p100/p10014354/81739927/81739927
- waves/p100/p10019003/87033314/87033314
- waves/p100/p10020306/83404654/83404654
- waves/p100/p10039708/83411188/83411188
- waves/p100/p10039708/85583557/85583557
Note the formatting of these records:
- intermediate directory ('p100' in this case)
- subject identifier (e.g. 'p10014354')
- record identifier (e.g. '81739927'
Q: Can you print the names of the last five records?
Hint: in Python, the last five elements can be specified using '[-5:]'
Extract metadata for a record¶
Each record contains metadata stored in a header file, named “<record name>.hea
”
Specify the online directory containing a record’s data¶
# Specify the 4th record (note, in Python indexing begins at 0)
idx = 3
record = records[idx]
record_dir = f'{database_name}/{record.parent}'
print("PhysioNet directory specified for record: {}".format(record_dir))
PhysioNet directory specified for record: mimic4wdb/0.1.0/waves/p100/p10039708/83411188
Specify the subject identifier¶
Extract the record name (e.g. ‘83411188’) from the record (e.g. ‘p100/p10039708/83411188/83411188’):
record_name = record.name
print("Record name: {}".format(record_name))
Record name: 83411188
Load the metadata for this record¶
Use the
rdheader
function from the WFDB toolbox to load metadata from the record header file
record_data = wfdb.rdheader(record_name, pn_dir=record_dir, rd_segments=True)
remote_url = "https://physionet.org/content/" + record_dir + "/" + record_name + ".hea"
print(f"Done: metadata loaded for record '{record_name}' from the header file at:\n{remote_url}")
Done: metadata loaded for record '83411188' from the header file at:
https://physionet.org/content/mimic4wdb/0.1.0/waves/p100/p10039708/83411188/83411188.hea
Inspect details of physiological signals recorded in this record¶
Printing a few details of the signals from the extracted metadata
print(f"- Number of signals: {record_data.n_sig}".format())
print(f"- Duration: {record_data.sig_len/(record_data.fs*60*60):.1f} hours")
print(f"- Base sampling frequency: {record_data.fs} Hz")
- Number of signals: 6
- Duration: 14.2 hours
- Base sampling frequency: 62.4725 Hz
Inspect the segments making up a record¶
Each record is typically made up of several segments
segments = record_data.seg_name
print(f"The {len(segments)} segments from record {record_name} are:\n{segments}")
The 6 segments from record 83411188 are:
['83411188_0000', '83411188_0001', '83411188_0002', '83411188_0003', '83411188_0004', '83411188_0005']
The format of filename for each segment is: record directory, "_", segment number
Inspect an individual segment¶
Read the metadata for this segment¶
Read the metadata from the header file
seg_no = 2
segment_metadata = wfdb.rdheader(record_name=segments[seg_no], pn_dir=record_dir)
print(f"""Header metadata loaded for:
- the segment '{segment_metadata.record_name}'
- in record '{record_name}'
- for subject '{str(Path(record_dir).parent.parts[-1])}'
""")
Header metadata loaded for:
- the segment '83411188_0002'
- in record '83411188'
- for subject 'p10039708'
Find out what signals are present¶
print(f"This segment contains the following signals: {segment_metadata.sig_name}")
print(f"The signals are measured in units of: {segment_metadata.units}")
This segment contains the following signals: ['II', 'V', 'aVR', 'ABP', 'Pleth', 'Resp']
The signals are measured in units of: ['mV', 'mV', 'mV', 'mmHg', 'NU', 'Ohm']
See here for definitions of signal abbreviations.
Q: Which of these signals is no longer present in segment '83411188_0005'?
Find out how long each signal lasts¶
All signals in a segment are time-aligned, measured at the same sampling frequency, and last the same duration:
print(f"The signals have a base sampling frequency of {segment_metadata.fs:.1f} Hz")
print(f"and they last for {segment_metadata.sig_len/(segment_metadata.fs*60):.1f} minutes")
The signals have a base sampling frequency of 62.5 Hz
and they last for 0.9 minutes
Identify records suitable for analysis¶
The signals and their durations vary from one record (and segment) to the next.
Since most studies require specific types of signals (e.g. blood pressure and photoplethysmography signals), we need to be able to identify which records (or segments) contain the required signals and duration.
Setup¶
print(f"Earlier, we loaded {len(records)} records from the '{database_name}' database.")
Earlier, we loaded 200 records from the 'mimic4wdb/0.1.0' database.
Specify requirements¶
Required signals
required_sigs = ['ABP', 'Pleth']
Required duration
# convert from minutes to seconds
req_seg_duration = 10*60
Find out how many records meet the requirements¶
NB: This step may take a while. The results are copied below to save running it yourself.
matching_recs = {'dir':[], 'seg_name':[], 'length':[]}
for record in records:
print('Record: {}'.format(record), end="", flush=True)
record_dir = f'{database_name}/{record.parent}'
record_name = record.name
print(' (reading data)')
record_data = wfdb.rdheader(record_name,
pn_dir=record_dir,
rd_segments=True)
# Check whether the required signals are present in the record
sigs_present = record_data.sig_name
if not all(x in sigs_present for x in required_sigs):
print(' (missing signals)')
continue
# Get the segments for the record
segments = record_data.seg_name
# Check to see if the segment is 10 min long
# If not, move to the next one
gen = (segment for segment in segments if segment != '~')
for segment in gen:
print(' - Segment: {}'.format(segment), end="", flush=True)
segment_metadata = wfdb.rdheader(record_name=segment,
pn_dir=record_dir)
seg_length = segment_metadata.sig_len/(segment_metadata.fs)
if seg_length < req_seg_duration:
print(f' (too short at {seg_length/60:.1f} mins)')
continue
# Next check that all required signals are present in the segment
sigs_present = segment_metadata.sig_name
if all(x in sigs_present for x in required_sigs):
matching_recs['dir'].append(record_dir)
matching_recs['seg_name'].append(segment)
matching_recs['length'].append(seg_length)
print(' (met requirements)')
# Since we only need one segment per record break out of loop
break
else:
print(' (long enough, but missing signal(s))')
print(f"A total of {len(matching_recs['dir'])} records met the requirements:")
# import pandas as pd
#df_matching_recs = pd.DataFrame(data=matching_recs)
#df_matching_recs.to_csv('matching_records.csv', index=False)
#p=1
Record: waves/p100/p10014354/81739927/81739927 (reading data)
(missing signals)
Record: waves/p100/p10019003/87033314/87033314 (reading data)
(missing signals)
Record: waves/p100/p10020306/83404654/83404654 (reading data)
- Segment: 83404654_0000 (too short at 0.0 mins)
- Segment: 83404654_0001 (long enough, but missing signal(s))
- Segment: 83404654_0002 (too short at 0.1 mins)
- Segment: 83404654_0003 (too short at 0.3 mins)
- Segment: 83404654_0004 (long enough, but missing signal(s))
- Segment: 83404654_0005 (met requirements)
Record: waves/p100/p10039708/83411188/83411188 (reading data)
- Segment: 83411188_0000 (too short at 0.0 mins)
- Segment: 83411188_0001 (too short at 0.1 mins)
- Segment: 83411188_0002 (too short at 0.9 mins)
- Segment: 83411188_0003 (too short at 0.3 mins)
- Segment: 83411188_0004 (too short at 0.3 mins)
- Segment: 83411188_0005 (long enough, but missing signal(s))
Record: waves/p100/p10039708/85583557/85583557 (reading data)
(missing signals)
Record: waves/p100/p10079700/85594648/85594648 (reading data)
(missing signals)
Record: waves/p100/p10082591/84050536/84050536 (reading data)
(missing signals)
Record: waves/p101/p10100546/83268087/83268087 (reading data)
(missing signals)
Record: waves/p101/p10112163/88501826/88501826 (reading data)
(missing signals)
Record: waves/p101/p10126957/82924339/82924339 (reading data)
- Segment: 82924339_0000 (too short at 0.0 mins)
- Segment: 82924339_0001 (too short at 0.2 mins)
- Segment: 82924339_0002 (too short at 0.1 mins)
- Segment: 82924339_0003 (too short at 0.4 mins)
- Segment: 82924339_0004 (too short at 0.1 mins)
- Segment: 82924339_0005 (too short at 0.0 mins)
- Segment: 82924339_0006 (too short at 5.3 mins)
- Segment: 82924339_0007 (met requirements)
Record: waves/p102/p10209410/84248019/84248019 (reading data)
- Segment: 84248019_0000 (too short at 0.0 mins)
- Segment: 84248019_0001 (too short at 0.1 mins)
- Segment: 84248019_0002 (too short at 4.8 mins)
- Segment: 84248019_0003 (too short at 0.2 mins)
- Segment: 84248019_0004 (too short at 1.0 mins)
- Segment: 84248019_0005 (met requirements)
Record: waves/p103/p10303080/88399302/88399302 (reading data)
(missing signals)
Record: waves/p104/p10494990/88374538/88374538 (reading data)
(missing signals)
Record: waves/p105/p10560354/81105139/81105139 (reading data)
(missing signals)
Record: waves/p106/p10680081/86426168/86426168 (reading data)
(missing signals)
Record: waves/p108/p10882818/81826943/81826943 (reading data)
(missing signals)
Record: waves/p109/p10952189/82439920/82439920 (reading data)
- Segment: 82439920_0000 (too short at 0.0 mins)
- Segment: 82439920_0001 (too short at 0.1 mins)
- Segment: 82439920_0002 (too short at 0.0 mins)
- Segment: 82439920_0003 (too short at 0.1 mins)
- Segment: 82439920_0004 (met requirements)
Record: waves/p110/p11013146/82432904/82432904 (reading data)
(missing signals)
Record: waves/p111/p11109975/82800131/82800131 (reading data)
- Segment: 82800131_0000 (too short at 0.0 mins)
- Segment: 82800131_0001 (too short at 0.1 mins)
- Segment: 82800131_0002 (met requirements)
Record: waves/p113/p11320864/81312415/81312415 (reading data)
(missing signals)
Record: waves/p113/p11392990/84304393/84304393 (reading data)
- Segment: 84304393_0000 (too short at 0.0 mins)
- Segment: 84304393_0001 (met requirements)
Record: waves/p115/p11552552/82650378/82650378 (reading data)
(missing signals)
Record: waves/p115/p11552552/88733244/88733244 (reading data)
(missing signals)
Record: waves/p115/p11558369/88636340/88636340 (reading data)
(missing signals)
Record: waves/p115/p11573811/87942784/87942784 (reading data)
(missing signals)
Record: waves/p115/p11596691/86130599/86130599 (reading data)
(missing signals)
Record: waves/p116/p11699665/81602645/81602645 (reading data)
(missing signals)
Record: waves/p119/p11944882/88867862/88867862 (reading data)
- Segment: 88867862_0000 (too short at 0.0 mins)
- Segment: 88867862_0001 (long enough, but missing signal(s))
- Segment: 88867862_0002 (too short at 0.6 mins)
- Segment: 88867862_0003 (too short at 0.2 mins)
- Segment: 88867862_0004 (long enough, but missing signal(s))
- Segment: 88867862_0005 (too short at 6.5 mins)
- Segment: 88867862_0006 (too short at 2.6 mins)
- Segment: 88867862_0007 (long enough, but missing signal(s))
- Segment: 88867862_0008 (too short at 0.3 mins)
- Segment: 88867862_0009 (too short at 0.3 mins)
- Segment: 88867862_0010 (long enough, but missing signal(s))
- Segment: 88867862_0011 (long enough, but missing signal(s))
- Segment: 88867862_0012 (too short at 0.2 mins)
- Segment: 88867862_0013 (long enough, but missing signal(s))
- Segment: 88867862_0014 (too short at 0.0 mins)
- Segment: 88867862_0015 (too short at 0.1 mins)
- Segment: 88867862_0016 (too short at 0.2 mins)
- Segment: 88867862_0017 (too short at 0.0 mins)
- Segment: 88867862_0018 (too short at 0.1 mins)
- Segment: 88867862_0019 (too short at 0.1 mins)
- Segment: 88867862_0020 (long enough, but missing signal(s))
- Segment: 88867862_0021 (long enough, but missing signal(s))
- Segment: 88867862_0022 (long enough, but missing signal(s))
- Segment: 88867862_0023 (long enough, but missing signal(s))
- Segment: 88867862_0024 (long enough, but missing signal(s))
- Segment: 88867862_0025 (too short at 1.1 mins)
- Segment: 88867862_0026 (long enough, but missing signal(s))
- Segment: 88867862_0027 (long enough, but missing signal(s))
- Segment: 88867862_0028 (too short at 2.6 mins)
- Segment: 88867862_0029 (long enough, but missing signal(s))
- Segment: 88867862_0030 (too short at 0.1 mins)
- Segment: 88867862_0031 (long enough, but missing signal(s))
- Segment: 88867862_0032 (too short at 0.8 mins)
- Segment: 88867862_0033 (too short at 1.5 mins)
- Segment: 88867862_0034 (long enough, but missing signal(s))
- Segment: 88867862_0035 (long enough, but missing signal(s))
- Segment: 88867862_0036 (long enough, but missing signal(s))
- Segment: 88867862_0037 (too short at 0.7 mins)
- Segment: 88867862_0038 (long enough, but missing signal(s))
- Segment: 88867862_0039 (too short at 9.4 mins)
- Segment: 88867862_0040 (long enough, but missing signal(s))
- Segment: 88867862_0041 (long enough, but missing signal(s))
- Segment: 88867862_0042 (long enough, but missing signal(s))
- Segment: 88867862_0043 (long enough, but missing signal(s))
Record: waves/p121/p12168037/89464742/89464742 (reading data)
- Segment: 89464742_0000 (too short at 0.0 mins)
- Segment: 89464742_0001 (met requirements)
Record: waves/p121/p12173569/88958796/88958796 (reading data)
- Segment: 88958796_0000 (too short at 0.0 mins)
- Segment: 88958796_0001 (too short at 1.6 mins)
- Segment: 88958796_0002 (too short at 6.5 mins)
- Segment: 88958796_0003 (too short at 0.5 mins)
- Segment: 88958796_0004 (met requirements)
Record: waves/p121/p12188288/88995377/88995377 (reading data)
- Segment: 88995377_0000 (too short at 0.0 mins)
- Segment: 88995377_0001 (met requirements)
Record: waves/p122/p12226163/85982742/85982742 (reading data)
(missing signals)
Record: waves/p122/p12246674/81515145/81515145 (reading data)
(missing signals)
Record: waves/p122/p12290932/84513510/84513510 (reading data)
(missing signals)
Record: waves/p122/p12295067/86906600/86906600 (reading data)
(missing signals)
Record: waves/p124/p12408438/88826653/88826653 (reading data)
(missing signals)
Record: waves/p124/p12424398/81741333/81741333 (reading data)
- Segment: 81741333_0000 (too short at 0.0 mins)
- Segment: 81741333_0001 (long enough, but missing signal(s))
- Segment: 81741333_0002 (too short at 0.4 mins)
- Segment: 81741333_0003 (long enough, but missing signal(s))
- Segment: 81741333_0004 (too short at 0.4 mins)
- Segment: 81741333_0005 (too short at 0.1 mins)
- Segment: 81741333_0006 (too short at 1.1 mins)
- Segment: 81741333_0007 (long enough, but missing signal(s))
- Segment: 81741333_0008 (too short at 0.1 mins)
- Segment: 81741333_0009 (too short at 0.0 mins)
- Segment: 81741333_0010 (long enough, but missing signal(s))
- Segment: 81741333_0011 (too short at 0.1 mins)
- Segment: 81741333_0012 (too short at 0.0 mins)
- Segment: 81741333_0013 (too short at 0.0 mins)
- Segment: 81741333_0014 (too short at 0.1 mins)
- Segment: 81741333_0015 (long enough, but missing signal(s))
Record: waves/p124/p12449182/84179115/84179115 (reading data)
(missing signals)
Record: waves/p124/p12470697/84213440/84213440 (reading data)
(missing signals)
Record: waves/p125/p12545470/80674263/80674263 (reading data)
(missing signals)
Record: waves/p125/p12563752/85393521/85393521 (reading data)
(missing signals)
Record: waves/p125/p12567919/82552643/82552643 (reading data)
(missing signals)
Record: waves/p127/p12789108/85835247/85835247 (reading data)
(missing signals)
Record: waves/p128/p12801061/80154334/80154334 (reading data)
(missing signals)
Record: waves/p128/p12807320/81739815/81739815 (reading data)
(missing signals)
Record: waves/p128/p12847457/83054955/83054955 (reading data)
(missing signals)
Record: waves/p128/p12872596/85230771/85230771 (reading data)
- Segment: 85230771_0000 (too short at 0.0 mins)
- Segment: 85230771_0001 (too short at 0.1 mins)
- Segment: 85230771_0002 (too short at 0.3 mins)
- Segment: 85230771_0003 (too short at 0.1 mins)
- Segment: 85230771_0004 (met requirements)
Record: waves/p128/p12882754/84094519/84094519 (reading data)
(missing signals)
Record: waves/p129/p12933208/86643930/86643930 (reading data)
- Segment: 86643930_0000 (too short at 0.0 mins)
- Segment: 86643930_0001 (long enough, but missing signal(s))
- Segment: 86643930_0002 (too short at 0.1 mins)
- Segment: 86643930_0003 (long enough, but missing signal(s))
- Segment: 86643930_0004 (met requirements)
Record: waves/p129/p12961799/86114120/86114120 (reading data)
(missing signals)
Record: waves/p130/p13016481/81250824/81250824 (reading data)
- Segment: 81250824_0000 (too short at 0.0 mins)
- Segment: 81250824_0001 (too short at 1.3 mins)
- Segment: 81250824_0002 (too short at 0.1 mins)
- Segment: 81250824_0003 (too short at 0.0 mins)
- Segment: 81250824_0004 (too short at 1.4 mins)
- Segment: 81250824_0005 (met requirements)
Record: waves/p130/p13080738/85361504/85361504 (reading data)
(missing signals)
Record: waves/p130/p13090274/87473566/87473566 (reading data)
(missing signals)
Record: waves/p131/p13184012/87023605/87023605 (reading data)
(missing signals)
Record: waves/p132/p13208026/82342224/82342224 (reading data)
(missing signals)
Record: waves/p132/p13216117/81815063/81815063 (reading data)
(missing signals)
Record: waves/p132/p13240081/87706224/87706224 (reading data)
- Segment: 87706224_0000 (too short at 0.0 mins)
- Segment: 87706224_0001 (too short at 0.1 mins)
- Segment: 87706224_0002 (too short at 8.0 mins)
- Segment: 87706224_0003 (met requirements)
Record: waves/p133/p13335291/89017807/89017807 (reading data)
(missing signals)
Record: waves/p133/p13364831/82697127/82697127 (reading data)
(missing signals)
Record: waves/p133/p13386985/81002096/81002096 (reading data)
(missing signals)
Record: waves/p134/p13457656/88841356/88841356 (reading data)
(missing signals)
Record: waves/p134/p13496169/80200410/80200410 (reading data)
(missing signals)
Record: waves/p135/p13559368/86957236/86957236 (reading data)
(missing signals)
Record: waves/p136/p13620446/83988903/83988903 (reading data)
(missing signals)
Record: waves/p136/p13624686/83058614/83058614 (reading data)
- Segment: 83058614_0000 (too short at 0.0 mins)
- Segment: 83058614_0001 (too short at 0.1 mins)
- Segment: 83058614_0002 (too short at 0.1 mins)
- Segment: 83058614_0003 (too short at 0.0 mins)
- Segment: 83058614_0004 (too short at 0.0 mins)
- Segment: 83058614_0005 (met requirements)
Record: waves/p136/p13687171/82266835/82266835 (reading data)
(missing signals)
Record: waves/p137/p13724605/81178488/81178488 (reading data)
(missing signals)
Record: waves/p137/p13791821/82803505/82803505 (reading data)
- Segment: 82803505_0000 (too short at 0.0 mins)
- Segment: 82803505_0001 (too short at 0.1 mins)
- Segment: 82803505_0002 (long enough, but missing signal(s))
- Segment: 82803505_0003 (long enough, but missing signal(s))
- Segment: 82803505_0004 (long enough, but missing signal(s))
- Segment: 82803505_0005 (too short at 0.1 mins)
- Segment: 82803505_0006 (long enough, but missing signal(s))
- Segment: 82803505_0007 (too short at 3.8 mins)
- Segment: 82803505_0008 (long enough, but missing signal(s))
- Segment: 82803505_0009 (too short at 0.2 mins)
- Segment: 82803505_0010 (too short at 2.4 mins)
- Segment: 82803505_0011 (too short at 0.1 mins)
- Segment: 82803505_0012 (long enough, but missing signal(s))
- Segment: 82803505_0013 (too short at 0.2 mins)
- Segment: 82803505_0014 (long enough, but missing signal(s))
- Segment: 82803505_0015 (too short at 5.5 mins)
- Segment: 82803505_0016 (long enough, but missing signal(s))
- Segment: 82803505_0017 (met requirements)
Record: waves/p138/p13840756/85241465/85241465 (reading data)
(missing signals)
Record: waves/p139/p13909615/85874778/85874778 (reading data)
(missing signals)
Record: waves/p140/p14025287/86429079/86429079 (reading data)
(missing signals)
Record: waves/p140/p14043090/80351079/80351079 (reading data)
(missing signals)
Record: waves/p140/p14057060/81396664/81396664 (reading data)
(missing signals)
Record: waves/p140/p14071752/87613677/87613677 (reading data)
(missing signals)
Record: waves/p141/p14141512/85863370/85863370 (reading data)
(missing signals)
Record: waves/p141/p14191565/88574629/88574629 (reading data)
- Segment: 88574629_0000 (too short at 0.0 mins)
- Segment: 88574629_0001 (met requirements)
Record: waves/p142/p14260018/80493349/80493349 (reading data)
(missing signals)
Record: waves/p142/p14285792/87867111/87867111 (reading data)
- Segment: 87867111_0000 (too short at 0.0 mins)
- Segment: 87867111_0001 (long enough, but missing signal(s))
- Segment: 87867111_0002 (too short at 0.1 mins)
- Segment: 87867111_0003 (long enough, but missing signal(s))
- Segment: 87867111_0004 (too short at 1.0 mins)
- Segment: 87867111_0005 (long enough, but missing signal(s))
- Segment: 87867111_0006 (too short at 0.5 mins)
- Segment: 87867111_0007 (long enough, but missing signal(s))
- Segment: 87867111_0008 (long enough, but missing signal(s))
- Segment: 87867111_0009 (long enough, but missing signal(s))
- Segment: 87867111_0010 (too short at 0.1 mins)
- Segment: 87867111_0011 (long enough, but missing signal(s))
- Segment: 87867111_0012 (met requirements)
Record: waves/p143/p14328567/80030009/80030009 (reading data)
(missing signals)
Record: waves/p143/p14356077/84560969/84560969 (reading data)
- Segment: 84560969_0000 (too short at 0.0 mins)
- Segment: 84560969_0001 (met requirements)
Record: waves/p143/p14363499/87562386/87562386 (reading data)
- Segment: 87562386_0000 (too short at 0.0 mins)
- Segment: 87562386_0001 (met requirements)
Record: waves/p145/p14504374/89909100/89909100 (reading data)
(missing signals)
Record: waves/p145/p14573205/83993183/83993183 (reading data)
(missing signals)
Record: waves/p146/p14629329/89923227/89923227 (reading data)
(missing signals)
Record: waves/p146/p14678292/82257762/82257762 (reading data)
(missing signals)
Record: waves/p146/p14695840/88685937/88685937 (reading data)
- Segment: 88685937_0000 (too short at 0.0 mins)
- Segment: 88685937_0001 (met requirements)
Record: waves/p147/p14711488/80647461/80647461 (reading data)
(missing signals)
Record: waves/p147/p14739057/86201081/86201081 (reading data)
(missing signals)
Record: waves/p147/p14751132/87909383/87909383 (reading data)
(missing signals)
Record: waves/p147/p14759494/87555605/87555605 (reading data)
(missing signals)
Record: waves/p147/p14781701/80705205/80705205 (reading data)
(missing signals)
Record: waves/p147/p14782379/83682560/83682560 (reading data)
(missing signals)
Record: waves/p148/p14811560/88188377/88188377 (reading data)
(missing signals)
Record: waves/p149/p14931547/86120311/86120311 (reading data)
- Segment: 86120311_0000 (too short at 0.0 mins)
- Segment: 86120311_0001 (met requirements)
Record: waves/p150/p15030787/82582002/82582002 (reading data)
(missing signals)
Record: waves/p151/p15174162/89866183/89866183 (reading data)
- Segment: 89866183_0000 (too short at 0.0 mins)
- Segment: 89866183_0001 (too short at 0.2 mins)
- Segment: 89866183_0002 (too short at 0.1 mins)
- Segment: 89866183_0003 (too short at 0.0 mins)
- Segment: 89866183_0004 (too short at 3.0 mins)
- Segment: 89866183_0005 (too short at 0.0 mins)
- Segment: 89866183_0006 (too short at 0.1 mins)
- Segment: 89866183_0007 (too short at 0.0 mins)
- Segment: 89866183_0008 (too short at 0.1 mins)
- Segment: 89866183_0009 (too short at 0.2 mins)
- Segment: 89866183_0010 (too short at 0.1 mins)
- Segment: 89866183_0011 (too short at 0.0 mins)
- Segment: 89866183_0012 (too short at 0.0 mins)
- Segment: 89866183_0013 (too short at 0.0 mins)
- Segment: 89866183_0014 (met requirements)
Record: waves/p153/p15312343/89068160/89068160 (reading data)
- Segment: 89068160_0000 (too short at 0.0 mins)
- Segment: 89068160_0001 (too short at 1.3 mins)
- Segment: 89068160_0002 (met requirements)
Record: waves/p153/p15315368/83076714/83076714 (reading data)
(missing signals)
Record: waves/p153/p15342703/86380383/86380383 (reading data)
- Segment: 86380383_0000 (too short at 0.0 mins)
- Segment: 86380383_0001 (met requirements)
Record: waves/p154/p15450741/80222656/80222656 (reading data)
(missing signals)
Record: waves/p154/p15461634/89565005/89565005 (reading data)
(missing signals)
Record: waves/p154/p15465960/87710197/87710197 (reading data)
(missing signals)
Record: waves/p154/p15497782/80353107/80353107 (reading data)
(missing signals)
Record: waves/p155/p15524470/80713046/80713046 (reading data)
(missing signals)
Record: waves/p155/p15552902/85078610/85078610 (reading data)
- Segment: 85078610_0000 (too short at 0.0 mins)
- Segment: 85078610_0001 (too short at 0.1 mins)
- Segment: 85078610_0002 (too short at 0.1 mins)
- Segment: 85078610_0003 (too short at 0.0 mins)
- Segment: 85078610_0004 (too short at 2.1 mins)
- Segment: 85078610_0005 (too short at 0.0 mins)
- Segment: 85078610_0006 (too short at 0.0 mins)
- Segment: 85078610_0007 (too short at 1.2 mins)
- Segment: 85078610_0008 (met requirements)
Record: waves/p156/p15649186/87702634/87702634 (reading data)
- Segment: 87702634_0000 (too short at 0.0 mins)
- Segment: 87702634_0001 (too short at 0.1 mins)
- Segment: 87702634_0002 (too short at 0.1 mins)
- Segment: 87702634_0003 (too short at 0.9 mins)
- Segment: 87702634_0004 (too short at 0.1 mins)
- Segment: 87702634_0005 (too short at 8.6 mins)
- Segment: 87702634_0006 (too short at 0.1 mins)
- Segment: 87702634_0007 (met requirements)
Record: waves/p156/p15651262/87121214/87121214 (reading data)
(missing signals)
Record: waves/p156/p15685201/83226324/83226324 (reading data)
(missing signals)
Record: waves/p157/p15738617/84323120/84323120 (reading data)
(missing signals)
Record: waves/p158/p15855446/80620046/80620046 (reading data)
(missing signals)
Record: waves/p158/p15857793/84686667/84686667 (reading data)
- Segment: 84686667_0000 (too short at 0.0 mins)
- Segment: 84686667_0001 (too short at 1.5 mins)
- Segment: 84686667_0002 (met requirements)
Record: waves/p158/p15858229/85931717/85931717 (reading data)
(missing signals)
Record: waves/p158/p15859796/87162659/87162659 (reading data)
(missing signals)
Record: waves/p158/p15865327/84802706/84802706 (reading data)
- Segment: 84802706_0000 (too short at 0.0 mins)
- Segment: 84802706_0001 (too short at 0.0 mins)
- Segment: 84802706_0002 (met requirements)
Record: waves/p158/p15896656/81811182/81811182 (reading data)
- Segment: 81811182_0000 (too short at 0.0 mins)
- Segment: 81811182_0001 (too short at 0.1 mins)
- Segment: 81811182_0002 (too short at 0.0 mins)
- Segment: 81811182_0003 (too short at 0.1 mins)
- Segment: 81811182_0004 (met requirements)
Record: waves/p159/p15920699/84421559/84421559 (reading data)
- Segment: 84421559_0000 (too short at 0.0 mins)
- Segment: 84421559_0001 (too short at 5.3 mins)
- Segment: 84421559_0002 (too short at 0.1 mins)
- Segment: 84421559_0003 (too short at 0.0 mins)
- Segment: 84421559_0004 (too short at 0.1 mins)
- Segment: 84421559_0005 (met requirements)
Record: waves/p160/p16034243/88221516/88221516 (reading data)
- Segment: 88221516_0000 (too short at 0.0 mins)
- Segment: 88221516_0001 (too short at 3.0 mins)
- Segment: 88221516_0002 (too short at 0.0 mins)
- Segment: 88221516_0003 (too short at 0.1 mins)
- Segment: 88221516_0004 (too short at 4.0 mins)
- Segment: 88221516_0005 (too short at 0.3 mins)
- Segment: 88221516_0006 (too short at 5.2 mins)
- Segment: 88221516_0007 (met requirements)
Record: waves/p160/p16074364/84765423/84765423 (reading data)
(missing signals)
Record: waves/p160/p16089661/87257193/87257193 (reading data)
(missing signals)
Record: waves/p160/p16096029/87730914/87730914 (reading data)
(missing signals)
Record: waves/p162/p16215869/89184684/89184684 (reading data)
(missing signals)
Record: waves/p162/p16224237/84746493/84746493 (reading data)
(missing signals)
Record: waves/p163/p16331109/85937857/85937857 (reading data)
(missing signals)
Record: waves/p163/p16362820/85250800/85250800 (reading data)
(missing signals)
Record: waves/p164/p16442963/89328952/89328952 (reading data)
(missing signals)
Record: waves/p164/p16450343/87509018/87509018 (reading data)
(missing signals)
Record: waves/p165/p16547220/81902403/81902403 (reading data)
(missing signals)
Record: waves/p165/p16566444/80057524/80057524 (reading data)
- Segment: 80057524_0000 (too short at 0.0 mins)
- Segment: 80057524_0001 (long enough, but missing signal(s))
- Segment: 80057524_0002 (too short at 0.6 mins)
- Segment: 80057524_0003 (too short at 4.2 mins)
- Segment: 80057524_0004 (too short at 0.7 mins)
- Segment: 80057524_0005 (met requirements)
Record: waves/p165/p16587972/86803944/86803944 (reading data)
(missing signals)
Record: waves/p166/p16601928/88159741/88159741 (reading data)
(missing signals)
Record: waves/p166/p16644640/84209926/84209926 (reading data)
- Segment: 84209926_0000 (too short at 0.0 mins)
- Segment: 84209926_0001 (too short at 0.5 mins)
- Segment: 84209926_0002 (too short at 0.2 mins)
- Segment: 84209926_0003 (too short at 0.0 mins)
- Segment: 84209926_0004 (too short at 0.1 mins)
- Segment: 84209926_0005 (too short at 0.1 mins)
- Segment: 84209926_0006 (too short at 0.0 mins)
- Segment: 84209926_0007 (too short at 0.9 mins)
- Segment: 84209926_0008 (long enough, but missing signal(s))
- Segment: 84209926_0009 (too short at 0.3 mins)
- Segment: 84209926_0010 (too short at 1.3 mins)
- Segment: 84209926_0011 (too short at 0.1 mins)
- Segment: 84209926_0012 (too short at 0.0 mins)
- Segment: 84209926_0013 (too short at 0.0 mins)
- Segment: 84209926_0014 (too short at 0.1 mins)
- Segment: 84209926_0015 (long enough, but missing signal(s))
- Segment: 84209926_0016 (too short at 4.7 mins)
- Segment: 84209926_0017 (long enough, but missing signal(s))
- Segment: 84209926_0018 (met requirements)
Record: waves/p166/p16645935/86666275/86666275 (reading data)
(missing signals)
Record: waves/p166/p16662288/83394240/83394240 (reading data)
(missing signals)
Record: waves/p166/p16683551/83349747/83349747 (reading data)
(missing signals)
Record: waves/p167/p16709726/83959636/83959636 (reading data)
- Segment: 83959636_0000 (too short at 0.0 mins)
- Segment: 83959636_0001 (too short at 0.0 mins)
- Segment: 83959636_0002 (too short at 0.1 mins)
- Segment: 83959636_0003 (too short at 6.3 mins)
- Segment: 83959636_0004 (too short at 1.1 mins)
- Segment: 83959636_0005 (too short at 0.9 mins)
- Segment: 83959636_0006 (too short at 3.1 mins)
- Segment: 83959636_0007 (too short at 1.7 mins)
- Segment: 83959636_0008 (too short at 0.1 mins)
- Segment: 83959636_0009 (too short at 0.0 mins)
- Segment: 83959636_0010 (met requirements)
Record: waves/p167/p16715341/89989722/89989722 (reading data)
- Segment: 89989722_0000 (too short at 0.0 mins)
- Segment: 89989722_0001 (too short at 0.1 mins)
- Segment: 89989722_0002 (too short at 2.3 mins)
- Segment: 89989722_0003 (too short at 2.0 mins)
- Segment: 89989722_0004 (too short at 9.6 mins)
- Segment: 89989722_0005 (too short at 0.9 mins)
- Segment: 89989722_0006 (too short at 2.9 mins)
- Segment: 89989722_0007 (too short at 0.3 mins)
- Segment: 89989722_0008 (too short at 0.5 mins)
- Segment: 89989722_0009 (too short at 6.1 mins)
- Segment: 89989722_0010 (too short at 0.3 mins)
- Segment: 89989722_0011 (too short at 0.6 mins)
- Segment: 89989722_0012 (too short at 0.4 mins)
- Segment: 89989722_0013 (too short at 4.2 mins)
- Segment: 89989722_0014 (too short at 1.2 mins)
- Segment: 89989722_0015 (too short at 3.0 mins)
- Segment: 89989722_0016 (met requirements)
Record: waves/p168/p16818396/89225487/89225487 (reading data)
- Segment: 89225487_0000 (too short at 0.0 mins)
- Segment: 89225487_0001 (too short at 0.1 mins)
- Segment: 89225487_0002 (too short at 7.4 mins)
- Segment: 89225487_0003 (too short at 0.1 mins)
- Segment: 89225487_0004 (too short at 0.1 mins)
- Segment: 89225487_0005 (too short at 0.4 mins)
- Segment: 89225487_0006 (too short at 0.1 mins)
- Segment: 89225487_0007 (met requirements)
Record: waves/p168/p16820602/82493254/82493254 (reading data)
(missing signals)
Record: waves/p168/p16846280/83877081/83877081 (reading data)
(missing signals)
Record: waves/p168/p16848565/84917468/84917468 (reading data)
(missing signals)
Record: waves/p169/p16949991/88967754/88967754 (reading data)
(missing signals)
Record: waves/p169/p16955095/82284982/82284982 (reading data)
(missing signals)
Record: waves/p169/p16971339/87311151/87311151 (reading data)
(missing signals)
Record: waves/p170/p17032851/84391267/84391267 (reading data)
- Segment: 84391267_0000 (too short at 0.0 mins)
- Segment: 84391267_0001 (met requirements)
Record: waves/p171/p17107892/83439223/83439223 (reading data)
(missing signals)
Record: waves/p172/p17229504/80889556/80889556 (reading data)
- Segment: 80889556_0000 (too short at 0.0 mins)
- Segment: 80889556_0001 (too short at 0.6 mins)
- Segment: 80889556_0002 (met requirements)
Record: waves/p173/p17301721/85250558/85250558 (reading data)
- Segment: 85250558_0000 (too short at 0.0 mins)
- Segment: 85250558_0001 (too short at 6.1 mins)
- Segment: 85250558_0002 (too short at 0.0 mins)
- Segment: 85250558_0003 (too short at 0.1 mins)
- Segment: 85250558_0004 (too short at 8.1 mins)
- Segment: 85250558_0005 (too short at 0.1 mins)
- Segment: 85250558_0006 (too short at 3.0 mins)
- Segment: 85250558_0007 (too short at 4.5 mins)
- Segment: 85250558_0008 (too short at 0.1 mins)
- Segment: 85250558_0009 (too short at 0.4 mins)
- Segment: 85250558_0010 (too short at 0.2 mins)
- Segment: 85250558_0011 (met requirements)
Record: waves/p173/p17325001/84567505/84567505 (reading data)
- Segment: 84567505_0000 (too short at 0.0 mins)
- Segment: 84567505_0001 (too short at 0.2 mins)
- Segment: 84567505_0002 (too short at 0.1 mins)
- Segment: 84567505_0003 (too short at 0.0 mins)
- Segment: 84567505_0004 (too short at 0.4 mins)
- Segment: 84567505_0005 (met requirements)
Record: waves/p174/p17413562/88976763/88976763 (reading data)
(missing signals)
Record: waves/p174/p17459141/80111237/80111237 (reading data)
(missing signals)
Record: waves/p174/p17488284/84486962/84486962 (reading data)
- Segment: 84486962_0000 (too short at 0.0 mins)
- Segment: 84486962_0001 (too short at 3.6 mins)
- Segment: 84486962_0002 (too short at 0.5 mins)
- Segment: 84486962_0003 (too short at 0.2 mins)
- Segment: 84486962_0004 (too short at 0.0 mins)
- Segment: 84486962_0005 (too short at 0.1 mins)
- Segment: 84486962_0006 (too short at 0.4 mins)
- Segment: 84486962_0007 (too short at 0.3 mins)
- Segment: 84486962_0008 (too short at 0.5 mins)
- Segment: 84486962_0009 (long enough, but missing signal(s))
- Segment: 84486962_0010 (long enough, but missing signal(s))
- Segment: 84486962_0011 (long enough, but missing signal(s))
- Segment: 84486962_0012 (long enough, but missing signal(s))
- Segment: 84486962_0013 (long enough, but missing signal(s))
- Segment: 84486962_0014 (long enough, but missing signal(s))
- Segment: 84486962_0015 (too short at 0.1 mins)
- Segment: 84486962_0016 (too short at 0.2 mins)
- Segment: 84486962_0017 (long enough, but missing signal(s))
- Segment: 84486962_0018 (long enough, but missing signal(s))
- Segment: 84486962_0019 (long enough, but missing signal(s))
- Segment: 84486962_0020 (long enough, but missing signal(s))
- Segment: 84486962_0021 (long enough, but missing signal(s))
- Segment: 84486962_0022 (too short at 0.2 mins)
- Segment: 84486962_0023 (long enough, but missing signal(s))
- Segment: 84486962_0024 (too short at 0.1 mins)
- Segment: 84486962_0025 (long enough, but missing signal(s))
- Segment: 84486962_0026 (long enough, but missing signal(s))
- Segment: 84486962_0027 (long enough, but missing signal(s))
- Segment: 84486962_0028 (too short at 0.1 mins)
- Segment: 84486962_0029 (too short at 0.9 mins)
- Segment: 84486962_0030 (long enough, but missing signal(s))
- Segment: 84486962_0031 (long enough, but missing signal(s))
- Segment: 84486962_0032 (long enough, but missing signal(s))
- Segment: 84486962_0033 (long enough, but missing signal(s))
Record: waves/p174/p17490822/85814172/85814172 (reading data)
- Segment: 85814172_0000 (too short at 0.0 mins)
- Segment: 85814172_0001 (too short at 2.1 mins)
- Segment: 85814172_0002 (too short at 0.4 mins)
- Segment: 85814172_0003 (long enough, but missing signal(s))
- Segment: 85814172_0004 (long enough, but missing signal(s))
- Segment: 85814172_0005 (too short at 0.4 mins)
- Segment: 85814172_0006 (long enough, but missing signal(s))
- Segment: 85814172_0007 (met requirements)
Record: waves/p175/p17549883/88582885/88582885 (reading data)
(missing signals)
Record: waves/p175/p17578102/83863589/83863589 (reading data)
(missing signals)
Record: waves/p177/p17701063/88788921/88788921 (reading data)
(missing signals)
Record: waves/p177/p17708869/84580526/84580526 (reading data)
(missing signals)
Record: waves/p177/p17738824/88884866/88884866 (reading data)
- Segment: 88884866_0000 (too short at 0.0 mins)
- Segment: 88884866_0001 (too short at 9.6 mins)
- Segment: 88884866_0002 (too short at 0.0 mins)
- Segment: 88884866_0003 (too short at 0.1 mins)
- Segment: 88884866_0004 (long enough, but missing signal(s))
- Segment: 88884866_0005 (met requirements)
Record: waves/p177/p17744715/80497954/80497954 (reading data)
- Segment: 80497954_0000 (too short at 0.0 mins)
- Segment: 80497954_0001 (too short at 0.5 mins)
- Segment: 80497954_0002 (too short at 0.1 mins)
- Segment: 80497954_0003 (too short at 0.0 mins)
- Segment: 80497954_0004 (too short at 0.5 mins)
- Segment: 80497954_0005 (too short at 0.1 mins)
- Segment: 80497954_0006 (too short at 0.0 mins)
- Segment: 80497954_0007 (too short at 0.3 mins)
- Segment: 80497954_0008 (too short at 0.1 mins)
- Segment: 80497954_0009 (too short at 0.0 mins)
- Segment: 80497954_0010 (too short at 5.5 mins)
- Segment: 80497954_0011 (too short at 0.1 mins)
- Segment: 80497954_0012 (met requirements)
Record: waves/p178/p17802132/86917206/86917206 (reading data)
(missing signals)
Record: waves/p178/p17818849/84786832/84786832 (reading data)
(missing signals)
Record: waves/p179/p17957832/80666640/80666640 (reading data)
- Segment: 80666640_0000 (too short at 0.0 mins)
- Segment: 80666640_0001 (too short at 3.2 mins)
- Segment: 80666640_0002 (too short at 0.2 mins)
- Segment: 80666640_0003 (too short at 0.0 mins)
- Segment: 80666640_0004 (too short at 0.4 mins)
- Segment: 80666640_0005 (too short at 0.1 mins)
- Segment: 80666640_0006 (too short at 0.0 mins)
- Segment: 80666640_0007 (too short at 1.5 mins)
- Segment: 80666640_0008 (too short at 1.7 mins)
- Segment: 80666640_0009 (too short at 0.1 mins)
- Segment: 80666640_0010 (too short at 0.0 mins)
- Segment: 80666640_0011 (too short at 0.1 mins)
- Segment: 80666640_0012 (too short at 0.1 mins)
- Segment: 80666640_0013 (too short at 1.5 mins)
- Segment: 80666640_0014 (met requirements)
Record: waves/p179/p17973277/83801243/83801243 (reading data)
- Segment: 83801243_0000 (too short at 0.0 mins)
- Segment: 83801243_0001 (too short at 0.6 mins)
- Segment: 83801243_0002 (too short at 0.2 mins)
- Segment: 83801243_0003 (too short at 4.0 mins)
- Segment: 83801243_0004 (too short at 0.1 mins)
- Segment: 83801243_0005 (too short at 0.0 mins)
- Segment: 83801243_0006 (too short at 0.9 mins)
- Segment: 83801243_0007 (long enough, but missing signal(s))
- Segment: 83801243_0008 (too short at 8.2 mins)
- Segment: 83801243_0009 (long enough, but missing signal(s))
- Segment: 83801243_0010 (long enough, but missing signal(s))
- Segment: 83801243_0011 (too short at 0.8 mins)
- Segment: 83801243_0012 (long enough, but missing signal(s))
- Segment: 83801243_0013 (too short at 0.1 mins)
- Segment: 83801243_0014 (long enough, but missing signal(s))
- Segment: 83801243_0015 (too short at 0.1 mins)
- Segment: 83801243_0016 (long enough, but missing signal(s))
- Segment: 83801243_0017 (long enough, but missing signal(s))
- Segment: 83801243_0018 (too short at 0.1 mins)
- Segment: 83801243_0019 (long enough, but missing signal(s))
- Segment: 83801243_0020 (too short at 0.1 mins)
- Segment: 83801243_0021 (too short at 8.6 mins)
- Segment: 83801243_0022 (too short at 0.1 mins)
- Segment: 83801243_0023 (too short at 1.5 mins)
- Segment: 83801243_0024 (too short at 0.1 mins)
- Segment: 83801243_0025 (long enough, but missing signal(s))
- Segment: 83801243_0026 (too short at 0.1 mins)
- Segment: 83801243_0027 (long enough, but missing signal(s))
- Segment: 83801243_0028 (too short at 0.1 mins)
- Segment: 83801243_0029 (too short at 0.3 mins)
- Segment: 83801243_0030 (too short at 5.7 mins)
- Segment: 83801243_0031 (too short at 0.1 mins)
- Segment: 83801243_0032 (too short at 1.4 mins)
- Segment: 83801243_0033 (too short at 7.0 mins)
- Segment: 83801243_0034 (too short at 0.1 mins)
- Segment: 83801243_0035 (too short at 3.5 mins)
- Segment: 83801243_0036 (too short at 5.1 mins)
- Segment: 83801243_0037 (too short at 1.1 mins)
- Segment: 83801243_0038 (too short at 2.3 mins)
- Segment: 83801243_0039 (too short at 9.3 mins)
- Segment: 83801243_0040 (too short at 7.3 mins)
- Segment: 83801243_0041 (too short at 0.1 mins)
- Segment: 83801243_0042 (too short at 5.0 mins)
- Segment: 83801243_0043 (too short at 6.1 mins)
- Segment: 83801243_0044 (too short at 0.1 mins)
- Segment: 83801243_0045 (long enough, but missing signal(s))
- Segment: 83801243_0046 (too short at 0.4 mins)
- Segment: 83801243_0047 (too short at 0.5 mins)
- Segment: 83801243_0048 (too short at 0.1 mins)
- Segment: 83801243_0049 (too short at 0.3 mins)
- Segment: 83801243_0050 (too short at 4.4 mins)
- Segment: 83801243_0051 (long enough, but missing signal(s))
- Segment: 83801243_0052 (too short at 0.1 mins)
- Segment: 83801243_0053 (too short at 0.3 mins)
- Segment: 83801243_0054 (long enough, but missing signal(s))
- Segment: 83801243_0055 (too short at 0.1 mins)
- Segment: 83801243_0056 (long enough, but missing signal(s))
- Segment: 83801243_0057 (too short at 0.3 mins)
- Segment: 83801243_0058 (long enough, but missing signal(s))
- Segment: 83801243_0059 (too short at 0.1 mins)
- Segment: 83801243_0060 (too short at 0.2 mins)
- Segment: 83801243_0061 (too short at 0.1 mins)
- Segment: 83801243_0062 (too short at 0.9 mins)
- Segment: 83801243_0063 (long enough, but missing signal(s))
- Segment: 83801243_0064 (too short at 0.3 mins)
Record: waves/p180/p18011649/83973028/83973028 (reading data)
(missing signals)
Record: waves/p180/p18034077/88626898/88626898 (reading data)
(missing signals)
Record: waves/p180/p18080257/84939605/84939605 (reading data)
- Segment: 84939605_0000 (too short at 0.0 mins)
- Segment: 84939605_0001 (too short at 1.0 mins)
- Segment: 84939605_0002 (too short at 0.7 mins)
- Segment: 84939605_0003 (too short at 2.1 mins)
- Segment: 84939605_0004 (met requirements)
Record: waves/p181/p18109577/82141753/82141753 (reading data)
- Segment: 82141753_0000 (too short at 0.0 mins)
- Segment: 82141753_0001 (too short at 0.7 mins)
- Segment: 82141753_0002 (too short at 0.2 mins)
- Segment: 82141753_0003 (too short at 0.1 mins)
- Segment: 82141753_0004 (too short at 0.2 mins)
- Segment: 82141753_0005 (too short at 0.0 mins)
- Segment: 82141753_0006 (too short at 0.0 mins)
- Segment: 82141753_0007 (too short at 0.7 mins)
- Segment: 82141753_0008 (too short at 3.0 mins)
- Segment: 82141753_0009 (too short at 0.1 mins)
- Segment: 82141753_0010 (too short at 5.0 mins)
- Segment: 82141753_0011 (long enough, but missing signal(s))
- Segment: 82141753_0012 (long enough, but missing signal(s))
- Segment: 82141753_0013 (long enough, but missing signal(s))
- Segment: 82141753_0014 (too short at 3.3 mins)
- Segment: 82141753_0015 (long enough, but missing signal(s))
- Segment: 82141753_0016 (long enough, but missing signal(s))
- Segment: 82141753_0017 (too short at 0.3 mins)
- Segment: 82141753_0018 (met requirements)
Record: waves/p181/p18111015/86297093/86297093 (reading data)
(missing signals)
Record: waves/p181/p18195790/89743206/89743206 (reading data)
(missing signals)
Record: waves/p183/p18324626/86874920/86874920 (reading data)
- Segment: 86874920_0000 (too short at 0.0 mins)
- Segment: 86874920_0001 (too short at 0.1 mins)
- Segment: 86874920_0002 (too short at 4.0 mins)
- Segment: 86874920_0003 (too short at 0.4 mins)
- Segment: 86874920_0004 (too short at 1.7 mins)
- Segment: 86874920_0005 (too short at 0.1 mins)
- Segment: 86874920_0006 (too short at 0.0 mins)
- Segment: 86874920_0007 (too short at 0.3 mins)
- Segment: 86874920_0008 (too short at 0.1 mins)
- Segment: 86874920_0009 (too short at 0.0 mins)
- Segment: 86874920_0010 (too short at 0.2 mins)
- Segment: 86874920_0011 (too short at 1.2 mins)
- Segment: 86874920_0012 (long enough, but missing signal(s))
- Segment: 86874920_0013 (too short at 0.9 mins)
- Segment: 86874920_0014 (met requirements)
Record: waves/p184/p18423403/84261835/84261835 (reading data)
(missing signals)
Record: waves/p184/p18455165/85888407/85888407 (reading data)
(missing signals)
Record: waves/p184/p18466737/89821554/89821554 (reading data)
(missing signals)
Record: waves/p185/p18573443/85263057/85263057 (reading data)
(missing signals)
Record: waves/p186/p18667552/81139702/81139702 (reading data)
(missing signals)
Record: waves/p187/p18700695/81937383/81937383 (reading data)
- Segment: 81937383_0000 (too short at 0.0 mins)
- Segment: 81937383_0001 (long enough, but missing signal(s))
- Segment: 81937383_0002 (too short at 0.3 mins)
- Segment: 81937383_0003 (too short at 3.0 mins)
- Segment: 81937383_0004 (too short at 0.8 mins)
- Segment: 81937383_0005 (too short at 6.3 mins)
- Segment: 81937383_0006 (too short at 0.2 mins)
- Segment: 81937383_0007 (too short at 0.5 mins)
- Segment: 81937383_0008 (too short at 1.6 mins)
- Segment: 81937383_0009 (too short at 0.3 mins)
- Segment: 81937383_0010 (too short at 6.3 mins)
- Segment: 81937383_0011 (too short at 0.2 mins)
- Segment: 81937383_0012 (too short at 0.3 mins)
- Segment: 81937383_0013 (long enough, but missing signal(s))
- Segment: 81937383_0014 (too short at 1.2 mins)
- Segment: 81937383_0015 (long enough, but missing signal(s))
- Segment: 81937383_0016 (too short at 2.5 mins)
- Segment: 81937383_0017 (too short at 0.1 mins)
- Segment: 81937383_0018 (too short at 0.6 mins)
- Segment: 81937383_0019 (too short at 1.5 mins)
- Segment: 81937383_0020 (too short at 1.6 mins)
- Segment: 81937383_0021 (long enough, but missing signal(s))
- Segment: 81937383_0022 (too short at 5.5 mins)
- Segment: 81937383_0023 (long enough, but missing signal(s))
- Segment: 81937383_0024 (too short at 0.1 mins)
- Segment: 81937383_0025 (long enough, but missing signal(s))
- Segment: 81937383_0026 (too short at 0.2 mins)
- Segment: 81937383_0027 (long enough, but missing signal(s))
- Segment: 81937383_0028 (long enough, but missing signal(s))
- Segment: 81937383_0029 (long enough, but missing signal(s))
- Segment: 81937383_0030 (too short at 1.5 mins)
- Segment: 81937383_0031 (too short at 1.0 mins)
- Segment: 81937383_0032 (too short at 4.9 mins)
- Segment: 81937383_0033 (too short at 0.6 mins)
- Segment: 81937383_0034 (too short at 3.1 mins)
- Segment: 81937383_0035 (too short at 0.5 mins)
- Segment: 81937383_0036 (long enough, but missing signal(s))
- Segment: 81937383_0037 (too short at 0.1 mins)
- Segment: 81937383_0038 (long enough, but missing signal(s))
- Segment: 81937383_0039 (long enough, but missing signal(s))
- Segment: 81937383_0040 (too short at 0.6 mins)
- Segment: 81937383_0041 (too short at 6.7 mins)
- Segment: 81937383_0042 (too short at 0.3 mins)
- Segment: 81937383_0043 (long enough, but missing signal(s))
- Segment: 81937383_0044 (too short at 0.3 mins)
- Segment: 81937383_0045 (long enough, but missing signal(s))
- Segment: 81937383_0046 (long enough, but missing signal(s))
- Segment: 81937383_0047 (too short at 0.1 mins)
- Segment: 81937383_0048 (long enough, but missing signal(s))
- Segment: 81937383_0049 (too short at 0.2 mins)
- Segment: 81937383_0050 (long enough, but missing signal(s))
- Segment: 81937383_0051 (too short at 0.1 mins)
- Segment: 81937383_0052 (long enough, but missing signal(s))
- Segment: 81937383_0053 (too short at 4.4 mins)
Record: waves/p187/p18709273/88457020/88457020 (reading data)
(missing signals)
Record: waves/p187/p18742074/84505262/84505262 (reading data)
- Segment: 84505262_0000 (too short at 0.0 mins)
- Segment: 84505262_0001 (too short at 0.1 mins)
- Segment: 84505262_0002 (too short at 0.1 mins)
- Segment: 84505262_0003 (too short at 0.1 mins)
- Segment: 84505262_0004 (too short at 0.0 mins)
- Segment: 84505262_0005 (too short at 0.1 mins)
- Segment: 84505262_0006 (too short at 0.1 mins)
- Segment: 84505262_0007 (too short at 0.0 mins)
- Segment: 84505262_0008 (too short at 0.1 mins)
- Segment: 84505262_0009 (too short at 1.4 mins)
- Segment: 84505262_0010 (met requirements)
Record: waves/p187/p18768904/82650643/82650643 (reading data)
(missing signals)
Record: waves/p187/p18790258/85940419/85940419 (reading data)
(missing signals)
Record: waves/p187/p18790977/87554500/87554500 (reading data)
(missing signals)
Record: waves/p188/p18824975/86288257/86288257 (reading data)
- Segment: 86288257_0000 (too short at 0.0 mins)
- Segment: 86288257_0001 (met requirements)
Record: waves/p188/p18881392/85145725/85145725 (reading data)
(missing signals)
Record: waves/p189/p18981843/86164656/86164656 (reading data)
(missing signals)
Record: waves/p190/p19052042/84601191/84601191 (reading data)
(missing signals)
Record: waves/p191/p19126489/89699401/89699401 (reading data)
- Segment: 89699401_0000 (too short at 0.0 mins)
- Segment: 89699401_0001 (met requirements)
Record: waves/p192/p19290410/81362381/81362381 (reading data)
(missing signals)
Record: waves/p193/p19305085/87468061/87468061 (reading data)
(missing signals)
Record: waves/p193/p19313794/88537698/88537698 (reading data)
- Segment: 88537698_0000 (too short at 0.0 mins)
- Segment: 88537698_0001 (too short at 0.6 mins)
- Segment: 88537698_0002 (too short at 0.2 mins)
- Segment: 88537698_0003 (too short at 0.1 mins)
- Segment: 88537698_0004 (too short at 0.5 mins)
- Segment: 88537698_0005 (too short at 0.1 mins)
- Segment: 88537698_0006 (too short at 0.0 mins)
- Segment: 88537698_0007 (too short at 0.1 mins)
- Segment: 88537698_0008 (too short at 5.0 mins)
- Segment: 88537698_0009 (too short at 0.8 mins)
- Segment: 88537698_0010 (too short at 0.3 mins)
- Segment: 88537698_0011 (long enough, but missing signal(s))
- Segment: 88537698_0012 (too short at 6.7 mins)
- Segment: 88537698_0013 (met requirements)
Record: waves/p193/p19396598/82093805/82093805 (reading data)
(missing signals)
Record: waves/p194/p19477300/86239129/86239129 (reading data)
(missing signals)
Record: waves/p194/p19498855/81594095/81594095 (reading data)
(missing signals)
Record: waves/p195/p19504755/85059986/85059986 (reading data)
(missing signals)
Record: waves/p196/p19619764/83958172/83958172 (reading data)
- Segment: 83958172_0000 (too short at 0.0 mins)
- Segment: 83958172_0001 (met requirements)
Record: waves/p196/p19646228/87340466/87340466 (reading data)
(missing signals)
Record: waves/p196/p19664876/86315854/86315854 (reading data)
(missing signals)
Record: waves/p197/p19706392/89657546/89657546 (reading data)
(missing signals)
Record: waves/p197/p19772551/83530581/83530581 (reading data)
(missing signals)
Record: waves/p199/p19918916/89922194/89922194 (reading data)
(missing signals)
Record: waves/p199/p19979360/89865959/89865959 (reading data)
(missing signals)
Record: waves/p199/p19994379/87407093/87407093 (reading data)
(missing signals)
A total of 52 records met the requirements:
print(f"A total of {len(matching_recs['dir'])} out of {len(records)} records met the requirements.")
relevant_segments_names = "\n - ".join(matching_recs['seg_name'])
print(f"\nThe relevant segment names are:\n - {relevant_segments_names}")
relevant_dirs = "\n - ".join(matching_recs['dir'])
print(f"\nThe corresponding directories are: \n - {relevant_dirs}")
A total of 52 out of 200 records met the requirements.
The relevant segment names are:
- 83404654_0005
- 82924339_0007
- 84248019_0005
- 82439920_0004
- 82800131_0002
- 84304393_0001
- 89464742_0001
- 88958796_0004
- 88995377_0001
- 85230771_0004
- 86643930_0004
- 81250824_0005
- 87706224_0003
- 83058614_0005
- 82803505_0017
- 88574629_0001
- 87867111_0012
- 84560969_0001
- 87562386_0001
- 88685937_0001
- 86120311_0001
- 89866183_0014
- 89068160_0002
- 86380383_0001
- 85078610_0008
- 87702634_0007
- 84686667_0002
- 84802706_0002
- 81811182_0004
- 84421559_0005
- 88221516_0007
- 80057524_0005
- 84209926_0018
- 83959636_0010
- 89989722_0016
- 89225487_0007
- 84391267_0001
- 80889556_0002
- 85250558_0011
- 84567505_0005
- 85814172_0007
- 88884866_0005
- 80497954_0012
- 80666640_0014
- 84939605_0004
- 82141753_0018
- 86874920_0014
- 84505262_0010
- 86288257_0001
- 89699401_0001
- 88537698_0013
- 83958172_0001
The corresponding directories are:
- mimic4wdb/0.1.0/waves/p100/p10020306/83404654
- mimic4wdb/0.1.0/waves/p101/p10126957/82924339
- mimic4wdb/0.1.0/waves/p102/p10209410/84248019
- mimic4wdb/0.1.0/waves/p109/p10952189/82439920
- mimic4wdb/0.1.0/waves/p111/p11109975/82800131
- mimic4wdb/0.1.0/waves/p113/p11392990/84304393
- mimic4wdb/0.1.0/waves/p121/p12168037/89464742
- mimic4wdb/0.1.0/waves/p121/p12173569/88958796
- mimic4wdb/0.1.0/waves/p121/p12188288/88995377
- mimic4wdb/0.1.0/waves/p128/p12872596/85230771
- mimic4wdb/0.1.0/waves/p129/p12933208/86643930
- mimic4wdb/0.1.0/waves/p130/p13016481/81250824
- mimic4wdb/0.1.0/waves/p132/p13240081/87706224
- mimic4wdb/0.1.0/waves/p136/p13624686/83058614
- mimic4wdb/0.1.0/waves/p137/p13791821/82803505
- mimic4wdb/0.1.0/waves/p141/p14191565/88574629
- mimic4wdb/0.1.0/waves/p142/p14285792/87867111
- mimic4wdb/0.1.0/waves/p143/p14356077/84560969
- mimic4wdb/0.1.0/waves/p143/p14363499/87562386
- mimic4wdb/0.1.0/waves/p146/p14695840/88685937
- mimic4wdb/0.1.0/waves/p149/p14931547/86120311
- mimic4wdb/0.1.0/waves/p151/p15174162/89866183
- mimic4wdb/0.1.0/waves/p153/p15312343/89068160
- mimic4wdb/0.1.0/waves/p153/p15342703/86380383
- mimic4wdb/0.1.0/waves/p155/p15552902/85078610
- mimic4wdb/0.1.0/waves/p156/p15649186/87702634
- mimic4wdb/0.1.0/waves/p158/p15857793/84686667
- mimic4wdb/0.1.0/waves/p158/p15865327/84802706
- mimic4wdb/0.1.0/waves/p158/p15896656/81811182
- mimic4wdb/0.1.0/waves/p159/p15920699/84421559
- mimic4wdb/0.1.0/waves/p160/p16034243/88221516
- mimic4wdb/0.1.0/waves/p165/p16566444/80057524
- mimic4wdb/0.1.0/waves/p166/p16644640/84209926
- mimic4wdb/0.1.0/waves/p167/p16709726/83959636
- mimic4wdb/0.1.0/waves/p167/p16715341/89989722
- mimic4wdb/0.1.0/waves/p168/p16818396/89225487
- mimic4wdb/0.1.0/waves/p170/p17032851/84391267
- mimic4wdb/0.1.0/waves/p172/p17229504/80889556
- mimic4wdb/0.1.0/waves/p173/p17301721/85250558
- mimic4wdb/0.1.0/waves/p173/p17325001/84567505
- mimic4wdb/0.1.0/waves/p174/p17490822/85814172
- mimic4wdb/0.1.0/waves/p177/p17738824/88884866
- mimic4wdb/0.1.0/waves/p177/p17744715/80497954
- mimic4wdb/0.1.0/waves/p179/p17957832/80666640
- mimic4wdb/0.1.0/waves/p180/p18080257/84939605
- mimic4wdb/0.1.0/waves/p181/p18109577/82141753
- mimic4wdb/0.1.0/waves/p183/p18324626/86874920
- mimic4wdb/0.1.0/waves/p187/p18742074/84505262
- mimic4wdb/0.1.0/waves/p188/p18824975/86288257
- mimic4wdb/0.1.0/waves/p191/p19126489/89699401
- mimic4wdb/0.1.0/waves/p193/p19313794/88537698
- mimic4wdb/0.1.0/waves/p196/p19619764/83958172
Question: Is this enough data for a study? Consider different types of studies, e.g. assessing the performance of a previously proposed algorithm to estimate BP from the PPG signal, vs. developing a deep learning approach to estimate BP from the PPG.
Extract data for a segment¶
Provide a list of segments which meet the requirements for the study
segment_names = ['83404654_0005', '82924339_0007']
segment_dirs = ['mimic4wdb/0.1.0/waves/p100/p10020306/83404654',
'mimic4wdb/0.1.0/waves/p101/p10126957/82924339']
Specify a segment from which to extract data
rel_segment_no = 0
rel_segment_name = segment_names[rel_segment_no]
rel_segment_dir = segment_dirs[rel_segment_no]
print(f"Specified segment '{rel_segment_name}' in directory: '{rel_segment_dir}'")
Specified segment '83404654_0005' in directory: 'mimic4wdb/0.1.0/waves/p100/p10020306/83404654'
Extension: Have a look at the files which make up this record here (NB: you will need to scroll to the bottom of the page).
Use the
rdrecord
function from the WFDB toolbox to read the data for this segment.
segment_data = wfdb.rdrecord(record_name=rel_segment_name, pn_dir=rel_segment_dir)
print(f"Data loaded from segment: {rel_segment_name}")
Data loaded from segment: 83404654_0005
Look at class type of the object in which the data are stored:
print(f"Data stored in class of type: {type(segment_data)}")
Data stored in class of type: <class 'wfdb.io.record.Record'>
Resource: You can find out more about the class representing single segment WFDB records here.
Find out about the signals which have been extracted
print(f"This segment contains waveform data for the following {segment_data.n_sig} signals: {segment_data.sig_name}")
print(f"The signals are sampled at a base rate of {segment_data.fs} Hz (and some are sampled at multiples of this)")
print(f"They last for {segment_data.sig_len/(60*segment_data.fs):.1f} minutes")
This segment contains waveform data for the following 6 signals: ['II', 'V', 'aVR', 'ABP', 'Pleth', 'Resp']
The signals are sampled at a base rate of 62.4725 Hz (and some are sampled at multiples of this)
They last for 52.4 minutes
Question: Can you find out which signals are sampled at multiples of the base sampling frequency by looking at the following contents of the 'segment_data' variable?
vars(segment_data)
{'record_name': '83404654_0005',
'n_sig': 6,
'fs': 62.4725,
'counter_freq': 999.56,
'base_counter': 10219520.0,
'sig_len': 196480,
'base_time': None,
'base_date': None,
'comments': ['signal 0 (II): channel=0 bandpass=[0.5,35]',
'signal 1 (V): channel=1 bandpass=[0.5,35]',
'signal 2 (aVR): channel=2 bandpass=[0.5,35]'],
'sig_name': ['II', 'V', 'aVR', 'ABP', 'Pleth', 'Resp'],
'p_signal': array([[ 0.00000000e+00, -6.50000000e-02, -5.00000000e-03,
nan, 5.02929688e-01, 1.56120205e-01],
[ 5.00000000e-03, -4.50000000e-02, -5.00000000e-03,
nan, 5.02929688e-01, 1.56853164e-01],
[ 1.50000000e-02, -2.50000000e-02, 5.00000000e-03,
nan, 5.02929688e-01, 1.57097484e-01],
...,
[-1.50000000e-02, 7.00000000e-02, -4.00000000e-02,
7.25000000e+01, 5.74951172e-01, 3.57683850e-01],
[-1.50000000e-02, 5.50000000e-02, -4.50000000e-02,
7.25000000e+01, 5.70800781e-01, 3.61104324e-01],
[ 0.00000000e+00, 9.00000000e-02, -5.50000000e-02,
7.25000000e+01, 5.62255859e-01, 3.63791840e-01]]),
'd_signal': None,
'e_p_signal': None,
'e_d_signal': None,
'file_name': ['83404654_0005e.dat',
'83404654_0005e.dat',
'83404654_0005e.dat',
'83404654_0005p.dat',
'83404654_0005p.dat',
'83404654_0005r.dat'],
'fmt': ['516', '516', '516', '516', '516', '516'],
'samps_per_frame': [4, 4, 4, 2, 2, 1],
'skew': [None, None, None, None, None, None],
'byte_offset': [None, None, None, None, None, None],
'adc_gain': [200.0, 200.0, 200.0, 16.0, 4096.0, 4093.0],
'baseline': [8192, 8192, 8192, 800, 0, 2],
'units': ['mV', 'mV', 'mV', 'mmHg', 'NU', 'Ohm'],
'adc_res': [14, 14, 14, 13, 12, 12],
'adc_zero': [8192, 8192, 8192, 4096, 2048, 2048],
'init_value': [0, 0, 0, 0, 0, 0],
'checksum': [10167, 1300, 56956, 35887, 29987, 21750],
'block_size': [0, 0, 0, 0, 0, 0]}
Visualise the signals¶
Select a segment¶
Specify a segment from which to extract data
rel_segment_n = 1
rel_segment_name = segment_names[rel_segment_n]
rel_segment_dir = segment_dirs[rel_segment_n]
print(f"Specified segment '{rel_segment_name}' in directory: '{rel_segment_dir}'")
Specified segment '82924339_0007' in directory: 'mimic4wdb/0.1.0/waves/p101/p10126957/82924339'
Extract one minute of data from this segment¶
Specify the timings of the data to be extracted
# time since the start of the segment at which to begin extracting data
start_seconds = 20
n_seconds_to_load = 60
Find out the sampling frequency of the waveform data
segment_metadata = wfdb.rdheader(record_name=rel_segment_name,
pn_dir=rel_segment_dir)
print(f"Metadata loaded from segment: {rel_segment_name}")
fs = round(segment_metadata.fs)
Metadata loaded from segment: 82924339_0007
Extract the specified data
sampfrom = fs * start_seconds
sampto = fs * (start_seconds + n_seconds_to_load)
segment_data = wfdb.rdrecord(record_name=rel_segment_name,
sampfrom=sampfrom,
sampto=sampto,
pn_dir=rel_segment_dir)
print(f"{n_seconds_to_load} seconds of data extracted from segment {rel_segment_name}")
60 seconds of data extracted from segment 82924339_0007
Plot the extracted signals¶
Plot the extracted signals using the plot_wfdb function from the WFDB Toolbox.
title_text = f"Segment {rel_segment_name}"
wfdb.plot_wfdb(record=segment_data,
title=title_text,
time_units='seconds')
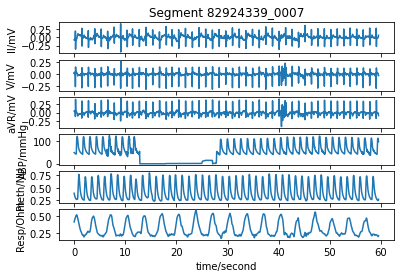
Extract the PPG signal to loook at it more closely
for sig_no in range(0, len(segment_data.sig_name)):
if "Pleth" in segment_data.sig_name[sig_no]:
break
ppg = segment_data.p_signal[:, sig_no]
fs = segment_data.fs
print(f"Extracted the PPG signal from column {sig_no} of the matrix of waveform data.")
Extracted the PPG signal from column 4 of the matrix of waveform data.
Note: the name given to PPG signals in the database is 'Pleth'.
Plot to look at the shape of the PPG pulse wave more closely
from matplotlib import pyplot as plt
import numpy as np
t = np.arange(0, (len(ppg) / fs), 1.0 / fs)
plt.plot(t, ppg, color = 'black', label='PPG')
plt.xlim([50, 55])
(50.0, 55.0)
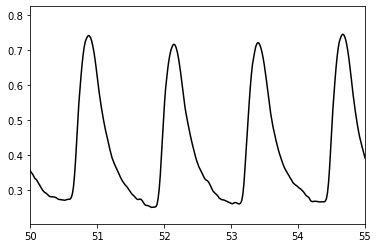
Compare this to pulse waves from the literature¶
Compare the pulse waves above to the different shapes of pulse waves shown here:
Source: Charlton PH et al., ‘Assessing hemodynamics from the photoplethysmogram to gain insights into vascular age: a review from VascAgeNet’, https://doi.org/10.1152/ajpheart.00392.2021 (CC BY 4.0)
These pulse waves are the typical shapes for young (class 1) to old (class 4) subjects.
Question: How do these pulse waves compare to those extracted from the MIMIC Database? Which is most similar?